Automated testing is essential in modern software development, ensuring quality, efficiency and scalability. As Agile and DevOps practices drive the demand for faster, more reliable testing processes, tools like Selenium and Python are at the forefront of automation testing.
This article covers the fundamentals of using Selenium with Python, including setup, scripting and leveraging advanced features. We’ll also highlight how T-Plan’s GUI-driven solutions enhance Selenium’s usability, making automation simpler and more accessible. By the end, you’ll have practical insights into the process, common challenges and reasons to consider T-Plan for your automation testing needs.
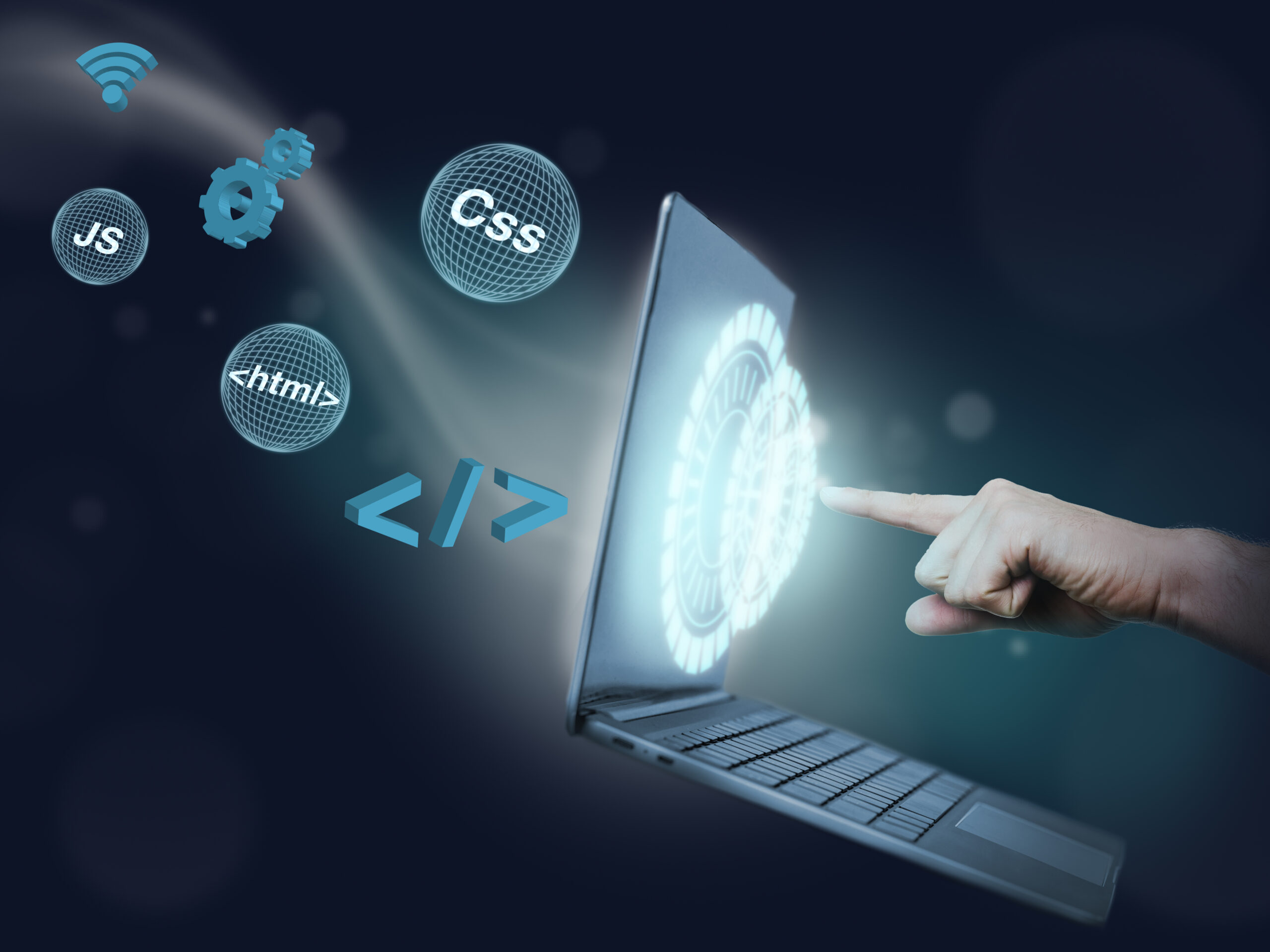
Table of Contents
Introduction to automated testing with Selenium and Python
What is Selenium?
Selenium is an open-source tool designed for automating web browsers. It offers unparalleled flexibility, cross-browser compatibility and support for multiple programming languages, including Java and JavaScript, making it a preferred choice for developers and testers alike.
Why pair Selenium with Python?
Python is an ideal companion for Selenium due to its simplicity, readability and rich ecosystem of libraries. Testers appreciate Python for its ability to quickly write scripts while benefiting from tools like pytest for test management and data processing libraries for deeper analysis.
Use cases: When to use Selenium and Python
From automating repetitive regression tests to performing complex cross-browser compatibility checks, Selenium and Python are versatile tools for:
- Validating form submissions and user workflows.
- Ensuring page elements render correctly across devices.
- Verifying the accuracy of APIs integrated with front-end components.
Enter T-Plan: Enhancing Selenium automation
While Selenium and Python offer incredible power, they demand a level of technical expertise that can slow teams down. This is where T-Plan excels. Our GUI-driven automation platform:
- Simplifies test creation: Build tests visually, without writing code.
- Minimises maintenance efforts: Intuitive interfaces adapt to changes, reducing manual updates.
- Extends functionality: Supports cross-platform testing, robust reporting and integration with test management tools.
Setting up Selenium and Python for testing
Installation and setup
To begin automating with Selenium and Python, ensure you have the following installed:
- Python: Download from Python.org and ensure it’s added to your system’s PATH.
- Selenium library: Use pip to install the selenium library.
- WebDriver: Obtain the browser-specific driver (e.g., ChromeDriver or GeckoDriver) compatible with your Selenium version.
For users on Windows, ensure all installations are correctly configured in your environment variables.
Challenges in manual setup
While these steps are straightforward for experienced users, configuring WebDrivers for multiple browsers and managing dependencies can be error-prone, especially when working across platforms.
T-Plan’s advantage
T-Plan eliminates setup headaches by offering a GUI-driven interface that automates configuration tasks. No more managing WebDriver versions or system paths – T-Plan handles it all, ensuring faster and smoother onboarding.
Key components of Selenium for web automation
Understanding Selenium WebDriver
At the heart of Selenium’s functionality lies WebDriver, the engine responsible for interacting with browsers. WebDriver communicates with your browser through native automation APIs, executing commands such as navigating to URLs, clicking elements or retrieving text. This powerful component is designed for:
- Cross-browser compatibility: Automate tests on browsers like Chrome, Firefox, Edge and Safari.
- Real user interaction simulation: Mimics mouse movements, keyboard inputs and other user actions for accurate testing.
- Flexibility: Easily integrates with other tools and frameworks, adapting to different test environments.
WebDriver’s reliability ensures that your tests run consistently across diverse browsers and systems, making it a cornerstone of any robust test automation strategy.
Locating and interacting with web elements
To automate interactions with a webpage, Selenium uses locators to identify elements within the DOM (Document Object Model). These locators ensure that your script can pinpoint specific elements, regardless of layout changes. Commonly used locators include:
- ID: Identifies elements with unique identifiers, such as id=”username”.
- Class name: Targets elements using class attributes, such as class=”login-button”.
- XPath: Defines complex or hierarchical paths to elements, useful for dynamic or nested components.
- CSS selectors: Provides flexibility for selecting elements using styles or attributes.
For example, a test that inputs a username and submits a form might look like this:
python
Copy code
from selenium import webdriver
driver = webdriver.Chrome()
driver.get(“https://example.com/login”)
# Locate and interact with web elements
username = driver.find_element_by_id(“username”)
username.send_keys(“test_user”)
submit_button = driver.find_element_by_class_name(“submit”)
submit_button.click()
driver.quit()
This snippet showcases how Selenium enables granular control over web interactions, ensuring precise automation.
Simplifying with T-Plan
While Selenium provides powerful capabilities, navigating the technical nuances of locators can be challenging, especially for beginners or teams without dedicated coding expertise. This is where T-Plan’s GUI-driven platform simplifies the process:
- Visual element selection: Eliminate the need to write XPath or CSS selectors manually by visually selecting elements on the interface.
- Intuitive automation: Define interactions like clicks or text inputs without diving into code.
- Improved efficiency: Reduce time spent troubleshooting locators and focus on validating test results.
With T-Plan, teams gain all the benefits of Selenium’s precision without the overhead of coding-heavy workflows.
Writing and running your first automated test
Example: Automating a login test
Creating your first Selenium test can be an exciting step in mastering automated testing. Below is an example of a basic script to automate the login process on a website:
python
Copy code
from selenium import webdriver
# Initialise the WebDriver
driver = webdriver.Chrome()
# Navigate to the login page
driver.get(“https://example.com/login”)
# Locate elements and perform actions
driver.find_element_by_id(“username”).send_keys(“test_user”)
driver.find_element_by_id(“password”).send_keys(“password123”)
driver.find_element_by_id(“submit”).click()
# Validate the result
assert “Dashboard” in driver.title, “Login failed or dashboard not loaded”
# Close the browser
driver.quit()
This script uses Selenium’s WebDriver to interact with the browser, simulating real user behaviour like entering a username and password, clicking a button and verifying the result.
Key concepts for writing Selenium tests
- Locating elements: Use strategies like find_element_by_id, find_element_by_class_name, or find_element_by_xpath to identify web elements accurately.
- Performing actions: Methods like send_keys() (for text input) and click() (for button presses) replicate user interactions.
- Assertions: Validate outcomes using Python’s assert statements, ensuring your test verifies the desired results.
Debugging and best practices
Testing with Selenium is a learning process, and starting with good practices will save time and effort in the long run.
- Start with simple tests: Focus on automating small, manageable workflows to understand the basics of Selenium and Python.
- Use browser developer tools: Inspect web elements to identify their locators. Tools like Chrome DevTools provide useful insights into IDs, class names and XPaths.
- Add logging and error handling: Incorporate logs to track test execution and error handling to manage unexpected behaviours.
Here’s an example of adding a logging statement:
python
Copy code
import logging
logging.basicConfig(level=logging.INFO)
logging.info(“Test execution started”)
driver = webdriver.Chrome()
driver.get(“https://example.com”)
logging.info(“Navigated to the login page”)
Visual test creation with T-Plan
For teams that want to reduce coding overhead or onboard new members quickly, T-Plan offers a visual approach to test creation:
- Drag-and-drop functionality: Build tests by dragging actions like clicks, text input or navigation onto a workflow.
- Code-free interaction mapping: Use T-Plan’s intuitive interface to define interactions without writing a single line of code.
- Instant feedback: Preview and execute tests directly within the platform, reducing iteration time.
This approach accelerates test creation while maintaining accuracy, enabling teams to focus on refining their testing strategy rather than troubleshooting scripts.
Advanced automation features in Selenium
As your automation journey progresses, you’ll encounter scenarios requiring more than basic interactions. From handling dynamic content to executing cross-browser tests, Selenium offers advanced capabilities to tackle these challenges effectively.
Handling dynamic content
Modern web applications frequently include dynamic elements – content that updates asynchronously or changes based on user interactions. Examples include pop-ups, dynamically loaded data and iframes.
Example: Waiting for a button to become clickable
Dynamic content often doesn’t load immediately, which can cause Selenium scripts to fail. To address this, Selenium provides explicit waits that pause execution until specific conditions are met. Here’s the script example:
python
Copy code
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Initialise WebDriver
driver = webdriver.Chrome()
# Wait for the button to become clickable
wait = WebDriverWait(driver, 10)
button = wait.until(EC.element_to_be_clickable((By.ID, “dynamic-button”)))
button.click()
Cross-browser testing with Selenium Grid
Testing across multiple browsers and devices ensures your application works seamlessly for all users. Selenium Grid enables parallel test execution, allowing you to run tests simultaneously on different environments. Some of the benefits of Selenium Grid include:
- Improved test coverage: Test on a wide range of browser and OS combinations.
- Faster execution: Reduce overall test execution time by running tests in parallel.
- Scalability: Add more nodes to the Grid as your testing needs grow.
Example setup for Selenium Grid:
To run tests on a remote node using Selenium Grid, update the WebDriver configuration to connect to the Grid hub:
python
Copy code
from selenium import webdriver
grid_url = “http://192.168.1.1:4444/wd/hub”
capabilities = {“browserName”: “chrome”}
driver = webdriver.Remote(command_executor=grid_url, desired_capabilities=capabilities)
driver.get(“https://example.com”)
print(driver.title)
driver.quit()
Setting up and maintaining a Grid infrastructure can be complex, requiring dedicated hardware or cloud resources.
How T-Plan simplifies advanced tasks
T-Plan eliminates the need for extensive coding and configuration by providing a GUI-driven interface for advanced automation features:
- Dynamic content handling: Built-in tools detect and interact with dynamic elements automatically, reducing the need for custom waits or scripts.
- Simplified cross-browser testing: Execute tests across browsers and platforms without the complexity of setting up Selenium Grid. T-Plan’s platform abstracts the infrastructure, letting you focus on test scenarios.
- Effortless iframe management: Visually select and switch between frames without writing manual code.
These capabilities allow teams to leverage Selenium’s advanced features with significantly reduced effort, accelerating test execution and improving reliability.
Integrating Selenium with Python testing frameworks
Popular frameworks for Selenium
Pytest is a lightweight and highly extensible testing framework. It is particularly suited for Selenium tests because of its support for:
- Parameterised testing: Run the same test with different inputs to validate multiple scenarios.
- Plugins: Extend functionality with plugins, such as pytest-html for generating test reports.
- Fixtures: Simplify setup and teardown processes, such as launching and closing browsers.
Unittest, Python’s built-in testing framework, provides a robust structure for organising test cases. Key features include:
- Test discovery: Automatically finds and executes test cases matching specific naming conventions.
- Assertions: Built-in methods for validating test outcomes, such as assertEqual and assertTrue.
- Test suites: Combine multiple tests into a suite for batch execution.
Framework benefits
Integrating Selenium with frameworks like pytest or unittest offers several advantages:
- Scalability: Manage thousands of test cases efficiently by grouping and organising them.
- Reusability: Share setup and teardown logic across tests, reducing duplication.
- Detailed reporting: Generate comprehensive test reports to track progress and identify failures.
- Error isolation: Run individual test cases to isolate and debug issues quickly.
How T-Plan enhances framework integration
T-Plan takes framework integration a step further by seamlessly supporting reporting, execution and test management. Key benefits include:
- Test management tools: Integrate with external reporting platforms for centralised tracking of test results.
- Simplified workflows: Execute tests and review results directly within T-Plan’s GUI, reducing reliance on command-line operations.
- Collaboration-friendly design: Share test reports and logs with team members effortlessly, streamlining feedback loops.
By combining T-Plan’s capabilities with frameworks like pytest or unittest, teams can achieve a well-rounded testing strategy, ensuring robust automation and simplified management.
Challenges of using Selenium for automation
While Selenium is a powerful tool for browser automation, its flexibility comes with certain challenges. These issues, if not addressed, can lead to inefficiencies and frustration during test development and execution. In this section, we’ll explore common pitfalls and how T-Plan helps overcome them.
Common issues
1. Flaky tests
Flaky tests are a persistent issue in automation, where test results vary between runs without changes to the application. Common causes include:
- Timing issues: Tests interacting with elements before they are fully loaded.
- Dynamic content: Elements that update asynchronously can lead to failures.
- Poor locator strategies: Over-reliance on brittle locators, such as XPaths tied to UI layouts.
2. Maintenance challenges
Applications evolve rapidly, with frequent updates to UI elements, workflows and functionalities. This requires constant updates to Selenium scripts to ensure:
- Locators are up-to-date.
- Test flows align with new workflows.
- Tests remain relevant for changed features.
3. Complexity
Selenium’s strength lies in its flexibility, but this can also make it challenging for non-technical users. Common complexities include:
- Writing and managing reusable code.
- Handling edge cases like pop-ups, file uploads or iframes.
- Setting up infrastructure like Selenium Grid for parallel testing.
Troubleshooting tips
Debugging flaky tests
Use explicit waits instead of hardcoded delays to ensure elements are ready before interacting:
python
Copy code
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
wait = WebDriverWait(driver, 10)
element = wait.until(EC.presence_of_element_located((By.ID, “dynamic-element”)))
element.click()
Improving locator strategies
- Prefer stable locators like IDs over XPaths tied to visual hierarchies.
- Use browser developer tools to inspect element attributes and select reliable identifiers.
Leveraging community support
The Selenium community is vast and active. Forums, GitHub repositories, and documentation provide invaluable resources for solving common problems.
How T-Plan addresses these challenges
1. Reducing flakiness
T-Plan mitigates flakiness with prebuilt, reliable solutions:
- Dynamic content handling: Automatically detect and wait for elements to load, reducing timing-related failures.
- Stable workflows: GUI-driven test creation abstracts locators, making tests resilient to minor UI changes.
2. Simplifying maintenance
T-Plan minimises maintenance overhead through intuitive visual tools:
- Visual editing: Easily update tests to reflect UI changes without modifying code.
- Centralised management: Track and update all test cases within a unified platform, ensuring consistency.
3. Making automation accessible
By eliminating the need for coding in many scenarios, T-Plan empowers non-technical users to contribute to automation efforts:
- Code-free test creation: Build tests visually with drag-and-drop actions.
- Simplified infrastructure: Cross-browser and parallel testing without complex Selenium Grid setups.
Why choose T-Plan for Selenium-based automation
Automated testing with Selenium and Python is a powerful approach to ensuring application quality, scalability and efficiency. However, as we’ve explored, the complexities of setup, script maintenance and advanced features can slow down teams and increase overhead.
This is where T-Plan offers a significant advantage. By integrating Selenium’s capabilities into a GUI-driven platform, T-Plan removes technical hurdles and accelerates test creation, especially for software testing teams. Some of the key takeaways include:
- Simplified workflows: T-Plan’s intuitive interface removes the need for extensive coding, making automation accessible to both technical and non-technical users.
- Enhanced stability: Features like dynamic content detection and prebuilt workflows reduce flakiness and minimise debugging efforts.
- Cross-platform testing: With out-of-the-box browser and OS support, T-Plan simplifies comprehensive cross-environment testing.
- Time and cost efficiency: Visual test creation and automated configurations drastically cut down on time spent maintaining and executing tests.
T-Plan is more than a tool – it’s a comprehensive solution designed to enhance every stage of your automation journey. By choosing T-Plan, your team can achieve:
- Faster test development with a reduced learning curve.
- Lower maintenance costs through visual test updates.
- Scalable, reliable test execution across diverse environments.
Start your journey with T-Plan
Take your Selenium-based automation to the next level with T-Plan. Whether you’re just starting with test automation or looking to optimise your existing processes, T-Plan offers the tools and insights you need to succeed.
Visit our website to:
- Read case studies from industry leaders.
- Contact us to discuss how we can help.
Achieve more with T-Plan – simplify your automation, deliver faster and ensure quality like never before.