Selenium WebDriver is a popular tool for automating web application testing on desktop and mobile platforms. It allows testers to interact with web applications just as a real user would. Comprehensive documentation accompanies Selenium WebDriver, which helps testers implement features correctly and troubleshoot effectively. By automating these integrations, Selenium WebDriver enables comprehensive testing of web applications and apps across different browsers, operating systems and platforms, ensuring consistency and reliability in functionality.
Being open-source, Selenium WebDriver is widely used and supported by a large community, which ensures regular updates and a wealth of resources for troubleshooting and optimisation. The robust capabilities of Selenium WebDriver make it an essential tool for any tester looking to streamline their web testing process, especially for regression testing and app testing, to ensure that new code changes do not introduce bugs into existing features.
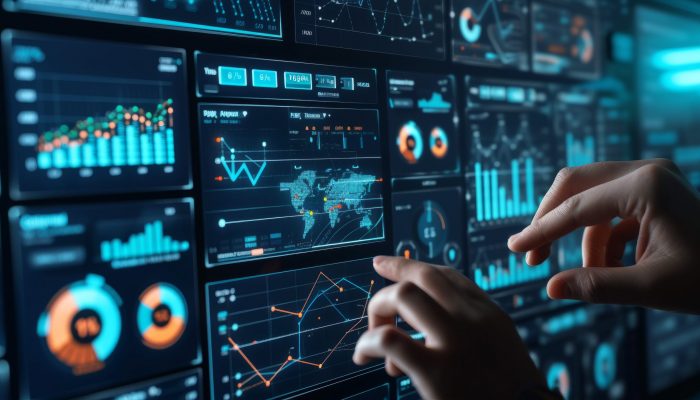
Table of Contents
Why browser automation testing is important
Efficiency: Automating repetitive tasks speeds up the testing process, allowing for quicker feedback and more efficient testing cycles.
Consistency: Automated tests reduce the risk of human error, providing consistent and repeatable results. This consistency is crucial for maintaining the integrity of the testing process, especially when simulating user interactions. By automating user interactions, you ensure that tests are executed in the same way every time, allowing you to reliably catch bugs and issues related to user interactions.
Faster release cycles: By integrating automated tests into the development pipeline, teams can achieve faster release cycles. This means that new features and bug fixes reach users more quickly, improving overall product quality and customer satisfaction. Selenium WebDriver supports rapid iteration and testing, which helps in accelerating the release cycles.
Setting Up Selenium WebDriver
Prerequisites and Installation
Required software (Java, Maven, browser drivers)
To get started with Selenium WebDriver, you’ll need to install several essential software components, such as the Java Development Kit (JDK) and browser drivers like ChromeDriver for desktop browsers.
- Java Development Kit (JDK): Selenium WebDriver is built on Java, so having the JDK installed is a prerequisite.
- Maven: This is a powerful project management tool that helps manage dependencies and build projects.
- Browser drivers: Depending on the browsers you intend to test on, you’ll need the corresponding browser drivers like ChromeDriver from Chrome, GeckoDriver for Firefox, etc.
Development environment setup
Setting up your development environment involves configuring your Integrated Development Environment (IDE) and ensuring all necessary plugins are installed. Here’s a step-by-step guide:
- Install JDK: Download and install the JDK from the official Oracle website. Set up the JAVA_HOME environment variable to point to the JDK installation directory.
- Install Maven: Download and install Maven. Set up the MAVEN_HOME environment variable and add Maven’s `bin` directory to your system’s PATH.
- Install an IDE: Popular IDEs like IntelliJ IDEA or Eclipse are highly recommended for Java development. Ensure the IDE is configured to recognise the JDK and Maven installations.
- Install necessary plugins: In your IDE, install any plugins necessary for Selenium development. For example, you might install plugins for Maven integration and browser driver management.
Downloading and configuring Selenium WebDriver
- Download Selenium WebDriver: You can download the Selenium WebDriver Java bindings from the official Selenium website. Add these to your Maven project’s `pom.xml` file as dependencies.
- Configure browser drivers: Download the appropriate browser drivers (e.g. ChromeDriver, GeckoDriver) and play them in a directory that is included in your system’s PATH.
- Write a basic script: Create a new Java project in your IDE, add the Selenium WebDriver dependencies, and write a basic script to test your setup. Here’s an example:
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class SeleniumTest { public static void main(String[] args) { System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver”); WebDriver driver = new ChromeDriver(); driver.get(“http://www.google.com”); System.out.println(“Title: ” + driver.getTitle()); driver.quit(); } } |
This script sets up a ChromeDriver instance, navigates to Google, prints the page title, and then closes the browser.
Basic Configuration
Setting up environment variables:
Instructions on how to set up environment variables for Selenium WebDriver to ensure smooth execution.
Example of a basic Selenium WebDriver script:
Provide a simple example script that demonstrates basic Selenium WebDriver functionalities such as launching a browser, navigating to a webpage, and performing basic interactions.
More advanced Selenium usage
Handling Different Browsers
Cross-browser testing
Cross-browser testing is essential to ensure that web applications function correctly across different browsers. Each browser interprets HTML, CSS, and JavaScript slightly differently, which can lead to inconsistencies in behaviour and appearance. By performing cross-browser testing, you can identify and fix these issues early in the development cycle.
Configuring tests for Chrome, Firefox, Edge, etc.:
To run Selenium tests on different browsers, you need to configure the respective browser drivers. Here are examples of how to set up tests for Chrome and Firefox:
Chrome:
System.setProperty(“webdriver.chrome.driver” , “path/to/chromedriver”); WebDriver driver = new ChromeDriver(); driver.get(“http://www.example.com”); |
Firefox:
System.setProperty(“webdriver.gecko.driver” , “path/to/geckodriver”); WebDriver driver = new FirefoxDriver(); driver.get(“http://www.example.com”); |
Similarly, you can configure tests for other browsers like Edge by downloading the respective driver and setting the system property accordingly.
Headless Browser Testing
Headless browser testing is a method where the browser operates without a graphical user interface (GUI). This type of testing is beneficial for scenarios where you need faster execution and do not require visual integration with the browser.
This method benefits from faster execution without the overhead of rendering the GUI, therefor tests run faster. It also consumes fewer system resources compared to traditional browser tests. However, there are still limitations. It is more challenging to debug tests as you cannot see the browser’ state visually, and some web applications might behave differently in headless mode.
Example setup for headless mode:
Here’s how to configure Chrome in headless mode:
System.setProperty(“webdriver.chrome.driver” , “path/to/chromedriver”); ChromeOptions options = new ChromeOptions(); options.addArguments(“headless”); WebDriver driver = new ChromeDriver(options); driver.get(“http://www.example.com”); |
Integration with Testing Frameworks
Using JUnit or TestNG with Selenium
Integrating Selenium with testing frameworks like JUnit or TestNG enhances your testing process by providing structure, better test management, and robust reporting capabilities. These frameworks allow you to organise your tests efficiently and run them in various configurations, such as sequentially, in parallel or conditionally based on test dependencies.
JUnit:
JUnit is a widely used testing framework for Java. It provides annotations and assertions that simplify the creation and management of test cases.
Example setup:
Add JUnit dependencies to your `pom.xml`:
<dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> <scope>test</scope> </dependency> |
Create simple Junit test:
import org.junit.Test; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import static org.junit.Assert.assertEquals; public class JUnitSeleniumTest { @Test public void testGoogleTitle() { System.setProperty(“webdriver.chrome.driver” , “path/to/chromedriver”); WebDriver driver = new ChromeDriver(options); driver.get(“http://www.google.com”); assertEquals(“Google”, driver.getTitle()); driver.quit(); } } |
This example shows a basic Junit test that launches Chrome, navigates to Google and verifies the page title.
Parallel execution in JUnit:
JUnit can be configured for parallel execution using the `@RunWith` and `@Suite` annotations. However, it requires additional setup and might be more straightforward in TestNG for complex parallel execution scenarios.
TestNG:
Add TestNG dependencies to your `pom.xml`:
<dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope> </dependency> |
Create simple Junit test:
import org.testng.annotations.Test; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import static org.testng.Assert.assertEquals; public class TestNGSeleniumTest { @Test public void testGoogleTitle() { System.setProperty(“webdriver.chrome.driver” , “path/to/chromedriver”); WebDriver driver = new ChromeDriver(options); driver.get(“http://www.google.com”); assertEquals(“Google”, driver.getTitle()); driver.quit(); } } |
This example demonstrates a basic TestNG test that performs similar actions to the Junit example.
Parallel execution in JUnit:
TestNG excels in parallel test execution, allowing you to run multiple tests concurrently. This is configured via the TESTNG XML configuration file.
<suite name=”Suite” parallel=”tests” thread-count=”2”> <test name=”Test1”> <classes> <class name=”com.example.TestNGSeleniumTest”/> </classes> </test> <test name=”Test2”> <classes> <class name=”com.example.TestNGSeleniumTest”/> </classes> </test> </suite> |
This configuration runs `Test1` and `Test2` in parallel, each using a separate thread.
Why use JUnit or TestNG with Selenium?
- Organised Test Management: Both frameworks provide a structured way to organise and manage your test cases, making it easier to maintain and scale your test suite.
- Annotations: Use annotations to define test methods, setup, teardown, and configuration methods, streamlining the test development process
- Assertions: Built-in assertion methods help validate test outcomes, making your tests more readable and reliable.
- Reporting: Generate detailed test reports that provide insights into test results, helping you identify issues and track test coverage.
- Parallel Execution: Run tests in parallel to reduce execution time and increase testing efficiency.
Leveraging Managed Testing Tools with Selenium
Using T-Plan Robot to streamline this process
T-Plan Robot is a versatile, managed testing tool that integrates seamlessly with Selenium WebDriver, providing additional capabilities and simplifying complex testing processes. T-Plan Robot offers an array of features such as advanced reporting, test management, and enhanced execution control – making it an ideal companion for Selenium WebDriver.
Integrating T-Plan Robot with Selenium WebDriver enhances your automation testing by providing a unified platform for managing and executing tests. This integration allows you to leverage the strengths of both tools, ensuring comprehensive coverage and robust automation.
Steps to Integrate T-Plan Robot with Selenium WebDriver:
- Configure T-Plan Robot for Selenium Integration: Open T-Plan Robot and navigate to the integration settings. Select Selenium WebDriver from the list of available integrations.
- Set Up Selenium WebDriver: Ensure you have Selenium WebDriver set up as described in the previous sections, including the necessary browser drivers and dependencies. Create a Selenium WebDriver script that performs the desired automation tasks.
- Crete a Test Case in T-Plan Robot: In T-Plan Robot, create a new test case and define the steps that correspond to your Selenium WebDriver script. Use T-Plan Robot’s scripting capabilities to call Selenium WebDriver commands within the test case.
Execute the test case in T-Plan Robot. T-Plan Robot will manage the execution of the Selenium WebDriver script, handle the browser interactions, and collect results.
As you can see this is far quicker and easier than coding test scripts yourself. Additionally, T-Plan offers exceptional technical and product support to help you achieve exactly what you need when using Selenium for automated browser testing.
This isn’t all it provides you with, however – T-Plan is a very comprehensive piece of software and also boasts all of the following features:
- Unified Reporting: T-Plan Robot consolidated results from Selenium WebDriver test with other test cases, providing comprehensive and unified reporting.
- Enhanced Test Management: Use T-Plan Robot’s advanced test management features to organise and schedule Selenium WebDriver tests alongside other automated and manual tests.
- Improved Execution Control: Leverage T-Plan Robot’s execution control features, such as parallel execution and distributed testing, to optimise test performance.
By integrating T-Plan Robot with Selenium WebDriver, you can create a powerful, flexible and efficient testing framework that takes advantage of the strengths of both tools, significantly enhancing your ability to manage and execute complex test scenarios, ensuring high-quality testing outcomes.
We offer a free demo of the T-Plan software, which you can get more information on using the link below.
Best Practices for Selenium Testing
Writing Maintainable Tests
Organising test code
Organising your test code effectively is crucial for maintaining and scaling your test suite. Here are some strategies:
- Use meaningful names: Use clear and descriptive names for your test classes and methods to indicate their purpose
- Follow a consistent structure: Organise your test files in a consistent structure, grouping related tests together. This could involve creating packages from different functional areas or types of tests (e.g. Smoke tests, regression tests).
- Separate test logic from setup/teardown: Use setup and teardown methods to handle tasks like opening and closing the browser. This keeps your test methods focused on the actual test logic.
Using Page Object Model (POM)
The Page Object Model is a design pattern that enhances test maintenance and reduces code duplication by creating an object repository for web elements.
Benefits of POM:
- Improved readability and maintainability: By separating the page elements and integrations from the test scripts, you make your tests more readable and easier to maintain.
- Code reusability: Page objects can be reused across different test cases, reducing duplication.
Example of a Page Object:
public class LoginPage { WebDriver driver; By username = By.id(“username”); By password = By.id(“password”); By loginButton = By.id(“loginBtn”); public LoginPage(WebDriver driver) { this.driver = driver; } public void setUsername(String user) { driver.findElement(username).sendKeys(user); } public void setPassword(String pass) { driver.findElement(password).sendKeys(pass); } public void clickLoginButton() { driver.findElement(loginButton).click(); } } |
Using the Page Object in a Test:
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.junit.Test; public class LoginTest { @Test public void testLogin() { System.setProperty(“webdriver.chrome.driver” , “path/to/chromedriver”); WebDriver driver = new ChromeDriver(); driver.get(“http://www.example.com/login”); LoginPage loginPage = new LoginPage (driver); loginPage.setUsername(“testuser”); loginPage.setPassword (“password”); loginPage.clickLoginButton(); // Add assertions here to verify login success driver.quit(); } } |
Debugging and Troubleshooting
Common issues and solutions:
- Element not found: Ensure that the locators used in your tests are correct and wait for elements to be present before interacting with them. Use explicit waits to handle dynamic content.
- Stale element reference: This occurs when an element is no longer attached to the DOM. Use retries or refetch the element to resolve this issue.
- Timeouts: Increase the implicit wait time or use explicit waits to give the elements enough time to load.
Using browser developer tools:
Browser developer tools can be very helpful in debugging Selenium tests. You can use them to:
- Inspect web elements to determine the correct locators.
- Monitor network requests to verify API calls and responses
- Check console logs for errors that might affect test execution.
Optimising Test Performance
Parallel testing strategies
Running tests in parallel is a powerful way to reduce the total time required for test execution. By executing multiple tests simultaneously, you can make better use of available resources and speed up the overall testing process. Both Junit and TestNG support parallel execution.
In JUnit you can configure your build too, such as Maven or Gradle, to run tests in parallel. This involves setting up the appropriate plugins and specifying the number of threads to be used. The configuration will vary depending on the build tool you use.
TestNG has built-in support for parallel test execution. You can configure the TestNG XML file to specify how tests should be run in parallel – whether at the level of methods, classes, or suites. By doing so, you can significantly decrease the time taken to complete the entire test suite.
The key to effective parallel execution is to ensure that your tests are independent of each other. This means that each test should set up its own test data and state and should not rely on the outcomes of other tests.
Efficient locator strategies
Creating efficient locators is key to reliable and fast test execution. Here are some tips:
- Use Unique IDs: Whenever possible use IDs to locate elements as they are the fastest and most reliable.
- Avoid absolute XPaths: Absolute XPaths are brittle and prone to break if the UI changes. Use relative XPaths or CSS selectors instead.
- Optimise CSS selections: Use specific and efficient CSS selectors to minimise the search scope.
Continuous Integration and Deployment (CI/CD)
Benefits of CI/CD in automated testing:
Continuous Integration (CI) and Continuous Deployment (CD) are essential practises in modern software development that help teams deliver high-quality software faster and more reliably. Integrating automated tests into the CI/CD pipeline offers several benefits:
Early Bug Detection: Automated tests run on every code change, allowing developers to catch and fix bugs early in the development process. This reduces the cost and effort required to address issues alter in the cycle.
Faster Feedback Loops: CI/CD pipelines provide quick feedback to developers, enabling them to make informed decisions and iterate rapidly. This accelerates the development process and helps maintain momentum.
Consistency and Reliability: Automated tests ensure that code changes are consistently verified against predefined criteria, leading to more reliable releases and higher confidence in the software’s quality.
Reduced Manual Effort: By automating repetitive testing tasks, teams can focus on more strategic activities, such as exploratory testing and improving test coverage.
Example setup with Jenkins and Docker:
Setting up a CI/CD pipeline with Jenkins and Docker can streamline the execution of Selenium tests. Here’s an overview of the process:
- Install Jenkins: Download and install Jenkins from the official website. Configure by installing necessary plugins, such as the Docker and Selenium plugins.
- Set Up Docker: Install Docker on your machine or use a Docker-compatible cloud service. Create Docker images for your application and Selenium tests, including all necessary dependencies and configurations.
- Create a Jenkins Pipeline: Define a Jenkins pipeline using a Jenkinsfile. This file describes the stages of your CI/CD process, including building the application, running tests and deploying the software. In the pipeline, use Docker to run your Selenium tests in isolated containers. This ensures consistency and prevents conflicts with the host environments.
Sample Jenkinsfile:
pipeline { agent any stages { stage(‘Build’) { steps { // Build your application Sh ‘docker build -t myapp .’ } } stage(‘Test’) { steps { // Run Selenium tests in Docker sh ‘docker run –rm myapp /bin.bash -c “mvn test” } } stage(‘Deploy’) { steps { //Deploy the application sh ‘cocker-compose up – d’ } } } post { always { //Clean up sh ‘docker-compose down’ } } } |
This Jenkinsfile defines a simple pipeline with build, test, and deploy stages. The `Test` stage runs the Selenium tests in a Docker container, ensuring a clean and consistent environment.
AI and machine learning in test automation:
The integration of AI and machine learning into test automation is an emerging trend that has the potential to revolutionise the way tests are created and executed. Here are some ways AI and machine learning can enhance test automation:
- Intelligent Test Generation: AI algorithms can analyse application behaviour and automatically generate test cases, reducing the manual effort required to create comprehensive test suites.
- Predictive Analytics: Machine learning models can predict areas of the application that are more likely to contain defects based on historical data, allowing teams to prioritise testing efforts effectively.
- Self-healing Tests: AI-driven tools can automatically update and replay test scripts when changes in the application break existing tests, reducing maintenance overhead.
- Enhanced Test Coverage: AI can identify gaps in test coverage and suggest additional test cases to ensure more thorough verification of the application.
By leveraging AI and machine learning, teams can achieve higher levels of efficiency, accuracy and coverage in the test automation efforts
Final Thoughts
Selenium WebDriver is a powerful tool for automating web application testing, and when combined with other tools and best practices it can significantly improve the efficiency and reliability of your testing process. By following the guidelines and techniques outlined in this article, you can build a robust and maintainable automation framework that meets the demands of modern software development.